0. repository 가 데이터를 접근하는 여러 방법들
1. entity
0. repository 가 데이터를 접근하는 여러 방법들
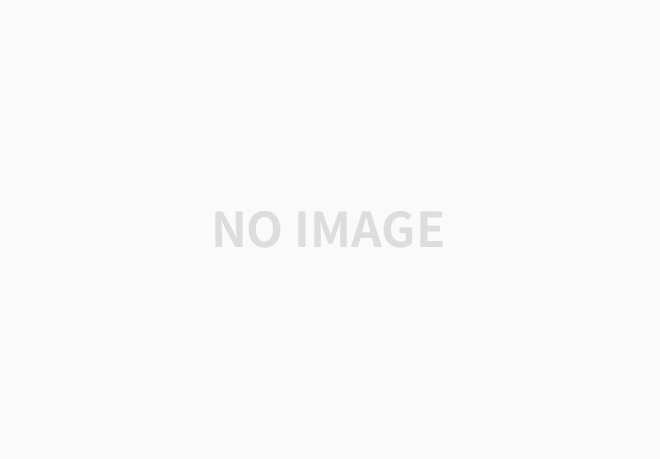
여러 시도를 해보기 위해서
각 entity 와 dto 클래스에
생성자와 메소드를 여러 개 만들어놨다.
Dto 클래스에 toEntity 메소드를 만드는 방법
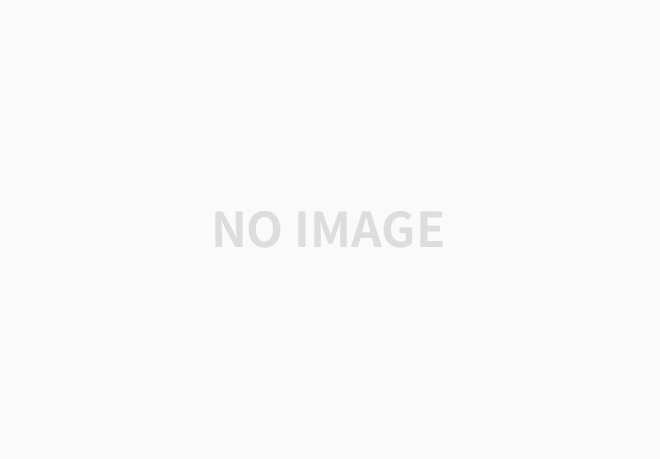
service 의 비즈니스 구현 메소드에서
dto -> entity 를 한 후, repository 가 db 로 접근하는방법이다.
Entity 클래스에서 toDto 메소드를 만드는 방법
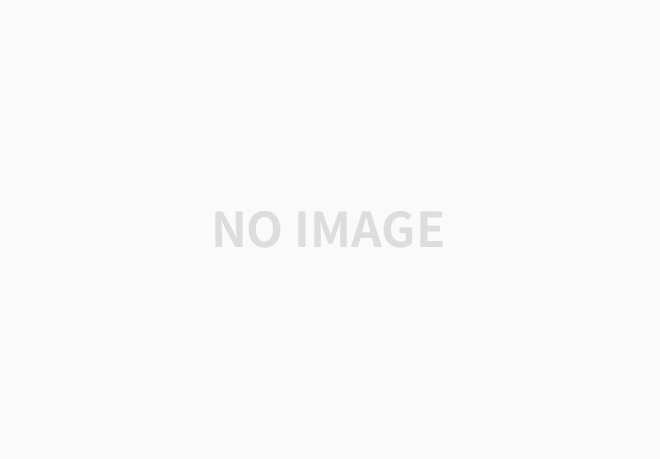
service 의 비즈니스 구현 메소드에서
repository 가 db 로 접근 + 데이터 조작 후에 dto 로 변환해주는 방법이다.
service 의 메소드에 파라미터로 데이터를 넣는 방법
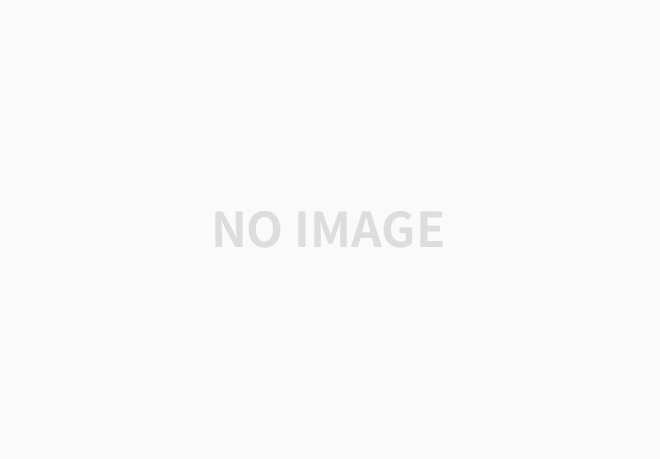
springSecurity 연습할 때 했던 방법인데,
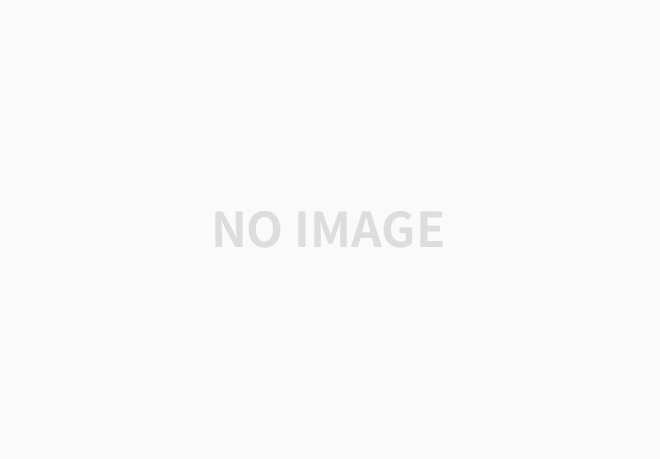
service 메소드를 이렇게 작성한 다음에, controller 에서
~~Dto.getTitle() 이렇게 작성하는 방법이다.
1. entity
DB 에 넣을 데이터를 정할 것이다.
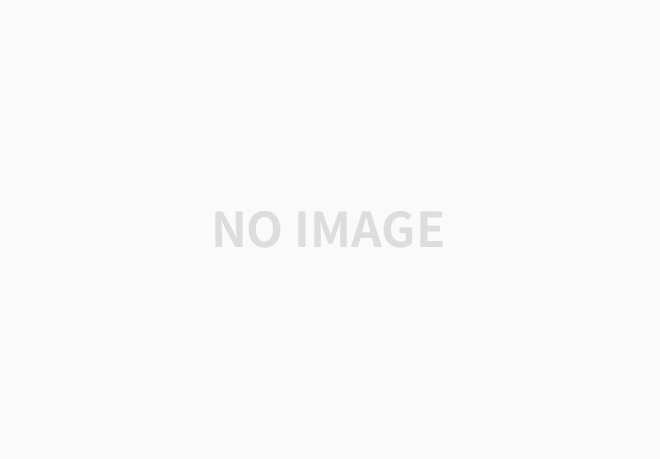
id: 글 id (기본키)
title: 글 제목
content: 글 내용
id: 이미지 id (기본키)
postEntity: 글 id (외래키)
imgName: 실제 로컬에 저장된 이미지 파일 이름
oriImgName: 업로드했던 이미지 파일 초기 이름
imgUrl: 업로드 결과 로컬에 저장된 이미지 파일을 불러올 경로
repimgYn: 대표이미지 여부
1-1 PostEntity
글 post 시 db 에 들어갈 entity
package com.example.blog.entity;
import com.example.blog.dto.PostDto;
import lombok.Data;
import lombok.NoArgsConstructor;
import javax.persistence.*;
@NoArgsConstructor
@Entity
@Table
@Data
public class PostEntity {
// id, title, content
@Id
@GeneratedValue
@Column(name="post_id")
private Long id;
private String title;
private String content;
// 글 수정 메소드
public void postPatch(PostEntity postEntity){
if(postEntity.title != null){
this.title = postEntity.title;
}
if(postEntity.content != null){
this.content = postEntity.content;
}
}
// 글+이미지 찾을 때 사용함
public PostDto toDto(){
return new PostDto(id,title, content);
}
}
DB 에 넣을 블로그 글 데이터이다.
글을 수정하기 위한 postPatch() 메소드를 선언해준다.
여러 시도를 했는데, 결국 toDto 방법이 제일 깔끔한 것 같다.
1-2 ImgEntity
package com.example.blog.entity;
import com.example.blog.dto.ImgDto;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import javax.persistence.*;
@Data
@Entity
@Table
@NoArgsConstructor
@AllArgsConstructor
public class ImgEntity {
@Id
@GeneratedValue
@Column(name="img_id")
private Long id;
private String imgName;
private String oriImgName;
private String imgUrl;
private String repimgYn; // 대표이미지 여부
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name="post_id")
private PostEntity postEntity;
public ImgEntity(Long id, String imgName, String oriImgName, String imgUrl, String repimgYn) {
this.id = id;
this.imgName = imgName;
this.oriImgName = oriImgName;
this.imgUrl = imgUrl;
this.repimgYn = repimgYn;
}
// for service
// public ImgEntity(String imgName, String oriImgName, String imgUrl) {
// this.imgName = imgName;
// this.oriImgName = oriImgName;
// this.imgUrl = imgUrl;
// }
// for service_patch_파라미터로 new entity 를 받음
// public void imgPatch(ImgEntity imgEntity){
// if(imgEntity.imgName != null){
// this.imgName = imgEntity.imgName;
// }
// if(imgEntity.oriImgName != null){
// this.oriImgName = imgEntity.oriImgName;
// }
// if(imgEntity.imgUrl != null){
// this.imgUrl = imgEntity.imgUrl;
// }
// }
// postImgService 에서 이미지 수정할 때 사용함
public void imgUpdate(String imgName, String oriImgName, String imgUrl){
this.imgName = imgName;
this.oriImgName = oriImgName;
this.imgUrl = imgUrl;
}
// 글+이미지 찾을 때 사용함
public ImgDto toDto(){
return new ImgDto(id,imgName,oriImgName,imgUrl,repimgYn);
}
}
DB 에 넣을 이미지 데이터이다.
주석처리 되어있는 메소드와 생성자는, Service 클래스에서 데이터 변경 시 사용하려 했었다.
외래키로 지정한 postEntity 변수이다.
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name="post_id")
private PostEntity postEntity;
@ManyToOne(fetch = FetchType.LAZY) 로 지연로딩 설정을 했다.
@JoinColumn(name="post_id") 로 PostEntity 테이블의 id 의 외래키로 지정했다.
댓글